RMI
Problem statement:
Design and implement client server application using RMI (Remote Method Invocation) to invoke a service to calculate the income tax.
Start NetBean IDE
Create new project:
File-> New Project
Select Java project and Click on next:
Provide the Project Name: (it-rmi-server)
And Click on Finish
Right click on package (it.rmi.server) Create new interface
Provide name for the interface: (ItTaxCal)
Click on Finish
package it.rmi.server;
import java.rmi.Remote;
public interface ItTaxCal extends Remote{
public interface ItTaxCal extends Remote{
}
Add the method to ItTaxCal interface as shown bellow:
package it.rmi.server;
import java.rmi.Remote;
import java.rmi.RemoteException;
public interface ItTaxCal extends Remote{
public double getTaxAmount(double income,double deductions)throws RemoteException;
}
Right Click on package and create new Class ( ItTaxCalImp)
Click on Finish
Note: Auto generate comments has been removed
Add the following code to the ItTaxCalImp class
package it.rmi.server;
import java.rmi.RemoteException;
import java.rmi.server.UnicastRemoteObject;
public class ItTaxCalImp extends UnicastRemoteObject implements ItTaxCal {
public ItTaxCalImp() throws RemoteException {
}
@Override
public double getTaxAmount(double income, double deductions) throws RemoteException {
//Write your Business Logic
double taxAmount = 0.0d;
if (income > deductions) {
taxAmount = (income - deductions) * .3;
}
return taxAmount;
}
}
Open ItRmiServer calss add the following code
package it.rmi.server;
import java.rmi.AlreadyBoundException;
import java.rmi.RemoteException;
import java.rmi.registry.LocateRegistry;
import java.rmi.registry.Registry;
public class ItRmiServer {
public static void main(String[] args) {
try{
Registry registry=LocateRegistry.createRegistry(1099);
ItTaxCal obj=new ItTaxCalImp();
registry.bind("taxcal", obj);
System.out.println("RMI Server is started......");
}catch(RemoteException | AlreadyBoundException e){
System.out.println(e);
}
}
}
With this we have done with the RMI server application
Creating client project:
Select Java Project and click on Next:
Provide project name and click on finish
To add Remote interface, right click on it-rmi-client project and select the Properties
Then the following window appears
Select the Libraries
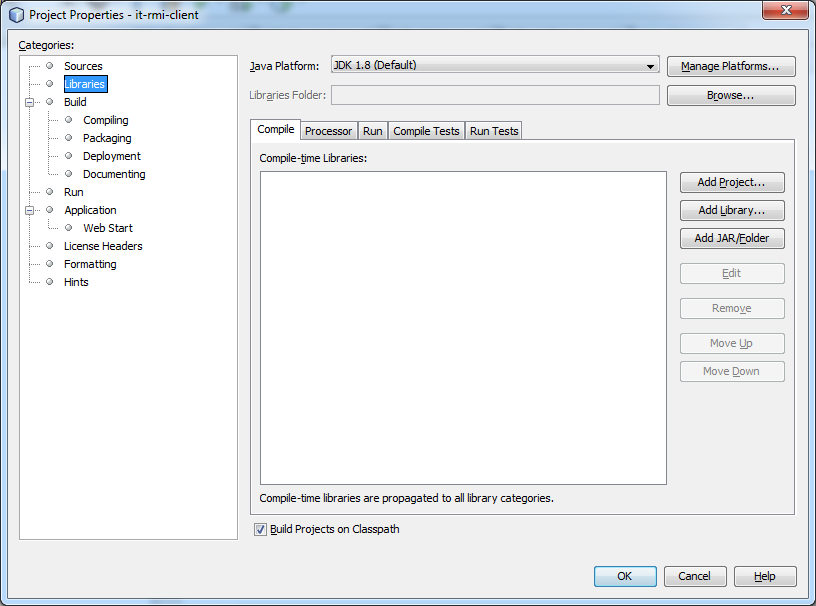
Select Add Project Tab, then select it-rmi-server project click on Add Project JAR Files
Open the ItRmiClient calss and add following code:
package it.rmi.client;
import it.rmi.server.ItTaxCal;
import java.rmi.NotBoundException;
import java.rmi.RemoteException;
import java.rmi.registry.LocateRegistry;
import java.rmi.registry.Registry;
import java.util.Scanner;
public class ItRmiClient {
public static void main(String[] args) {
try{
Registry registry=LocateRegistry.getRegistry("localhost",1099);
ItTaxCal obj=(ItTaxCal)registry.lookup("taxcal");
Scanner sc=new Scanner(System.in);
System.out.println("Enter your name:");
String name=sc.nextLine();
System.out.println("Enter Income :");
double income=sc.nextDouble();
System.out.println("Enter Deductions");
double deductions=sc.nextDouble();
double taxamt=obj.getTaxAmount(income, deductions);
System.out.println("\n Hi, "+name +" your tax details :");
System.out.println("Income :"+income);
System.out.println("Deductions :"+deductions);
System.out.println("Tax Amount :"+taxamt);
}catch(RemoteException | NotBoundException e){
System.out.println(e);
}
}
}
We are done with ItRmiClient class:
To Run the application:
First run the ItRmiServer Program (Open ItRmiServer)
Right click and RunFile
On console, RMI server is started…….
Now RMI server is running successfully. To access RMI Service we we should run client class
Open ItRmiClient class, Riht click and click on Run file
Based on your inputs tax calculation will be calculated and displayed on console.
No comments:
Post a Comment